DynamoDB localにはDynamoDB shellというサーバーが一緒に立ち上がってそこから操作できるようになっています。DynamoDB local立ち上げ後
http://localhost:8000/shell
でアクセスすると
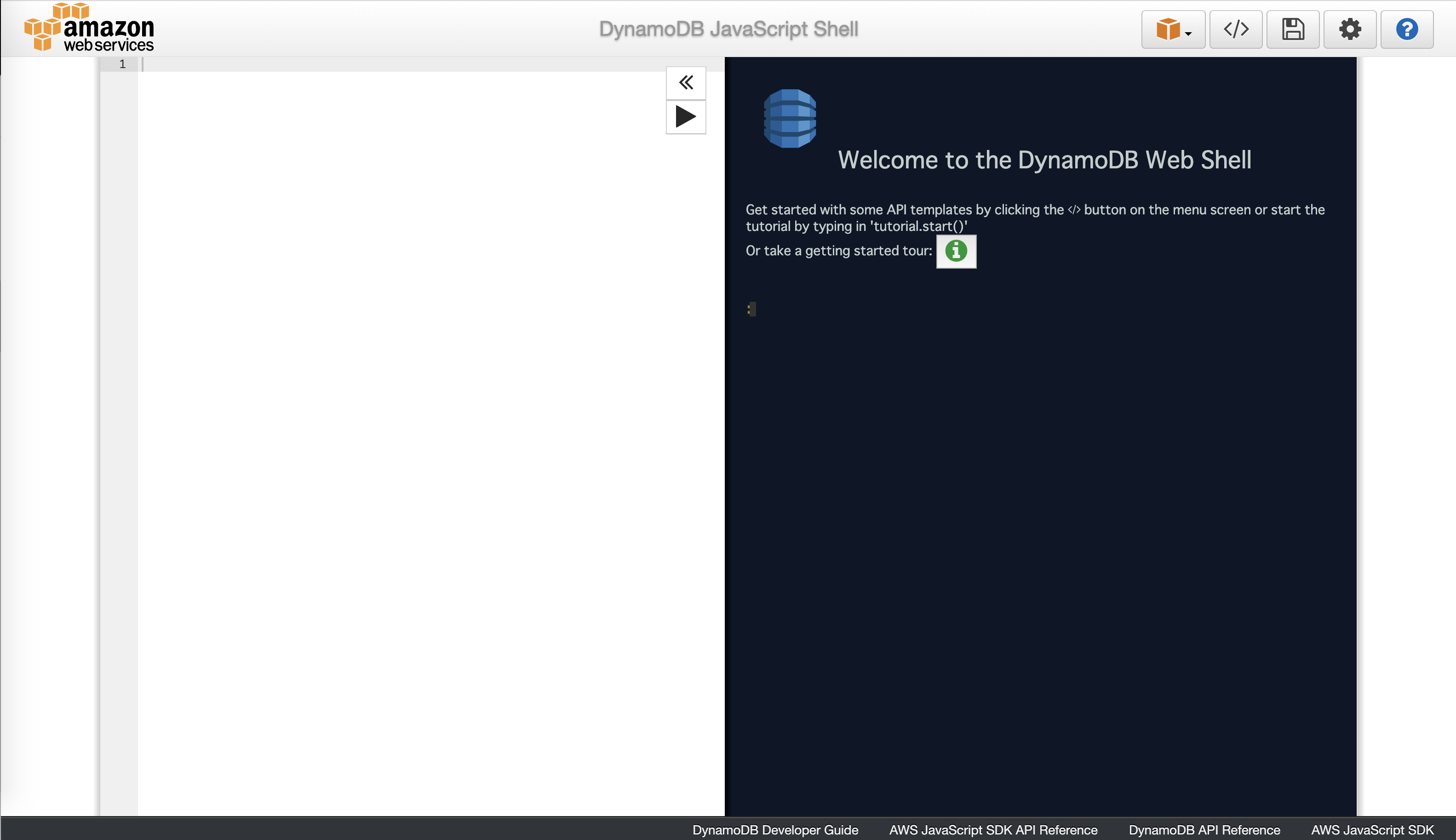
この様な画面が開けるので左側にコードを入力して▶を押すと実行結果が右側にでます。
dynamodbという変数にAWS.DynamoDB
のインスタンスが入っているのでそれを使用して実行していきます。
テーブル一覧を取得
dynamodb.listTables(null, (err, data) => {
if (err) {
console.log(err, err.stack)
} else {
console.log(data)
}
})
レスポンス
{"TableNames":["table0","table1","table2"]}
テーブルのスキーマ情報取得
dynamodb.describeTable({TableName: "tablename"}, (err, data) => {
if (err) {
console.log(err, err.stack)
} else {
console.log(data.Table.KeySchema)
}
})
レスポンス
[{"AttributeName":"user_id","KeyType":"HASH"},{"AttributeName":"created_at","KeyType":"RANGE"}]
HASHテーブル作成
dynamodb.createTable({
AttributeDefinitions: [{
AttributeName: 'hash_id',
AttributeType: 'N'
}],
KeySchema: [{
AttributeName: 'hash_id',
KeyType: 'HASH'
}],
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
},
TableName: 'hashtable',
StreamSpecification: {
StreamEnabled: false
}}, function(err, data) {
if (err) {
console.log(err, err.stack)
} else {
console.log(data)
}
})
複合(HASH&RANGE)テーブル作成
dynamodb.createTable({
AttributeDefinitions: [{
AttributeName: 'hash_id',
AttributeType: 'N'
},{
AttributeName: 'range_value',
AttributeType: 'S'
}],
KeySchema: [{
AttributeName: 'hash_id',
KeyType: 'HASH'
},{
AttributeName: 'range_value',
KeyType: 'RANGE'
}],
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
},
TableName: 'HashRangeTable',
StreamSpecification: {
StreamEnabled: false
}}, function(err, data) {
if (err) {
console.log("Error", err)
} else {
console.log("Table Created", data)
}
})
テーブル削除
dynamodb.deleteTable({TableName: "tablename"}, (err, data) => {
if (err) {
console.log(err, err.stack)
} else {
console.log(data)
}
})
レコードを取得
dynamodb.scan({TableName: "tablename"}, (err, res) => {
console.log(res)
})